Form validations in vehicle fleet management
Hello People. This article gives you information about Form validations in vehicle fleet management.
Imports
The react component needs to import various dependencies, including Mantine components and utilities for working with forms.
import React, { createContext, useContext, useMemo } from "react";
import { TbCalendar } from "react-icons/tb";
import {
MultiSelect,
Radio,
SegmentedControl,
SegmentedControlItem,
Select,
SelectItem,
Switch,
Text,
TextInput,
} from "@mantine/core";
import { DatePicker, DateRangePicker } from "@mantine/dates";
import { useForm, UseFormReturnType } from "@mantine/form";
import { FormValidateInput } from "@mantine/form/lib/types";
import { AutocompleteField } from "./components";
type ValidationFunction = (
value: unknown
) =>
| string
| number
| true
| React.ReactFragment
| React.ReactElement<any, string | React.JSXElementConstructor>
| null;
// ... (Other types and interfaces)
Types
It defines types such as ValidationFunction
, FieldConfig
, FormProps
, and FormGeneratorContextType
to specify the structure of form fields and their configurations.
For example:
type ValidationFunction = (
value: unknown
) =>
| string
| number
| true
| React.ReactFragment
| React.ReactElement<any, string | React.JSXElementConstructor>
| null;
export interface FieldConfig {
// ... (FieldConfig properties)
}
interface FormProps {
// ... (FormProps properties)
}
interface FormGeneratorContextType extends Omit<FormProps, "children" | "onSubmit"> {
form: UseFormReturnType<{}, (values: {}) => {}>;
}
// ... (FormContext and Form component definitions)
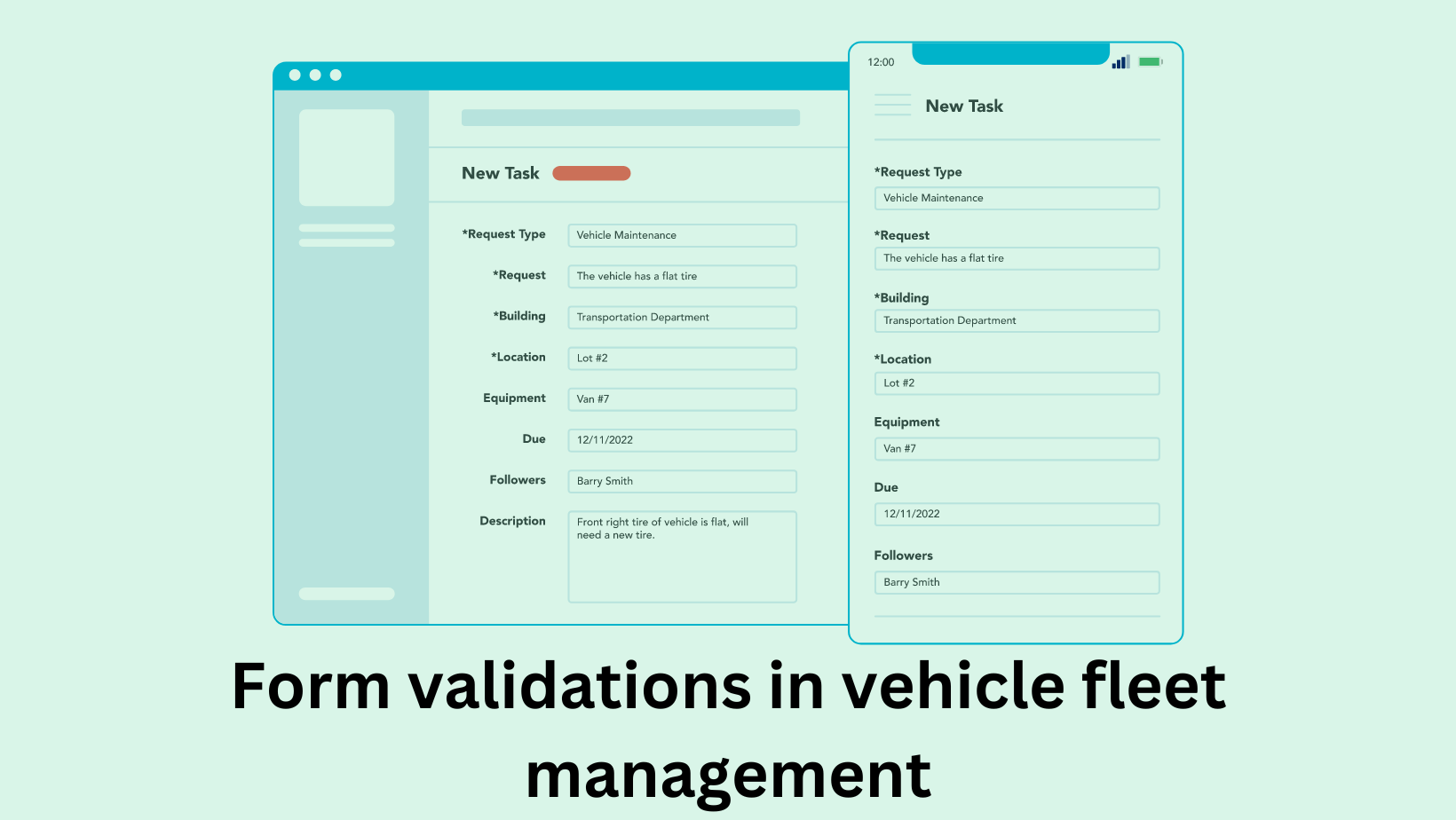
Context
It creates a React context (FormContext
) to manage and share form-related information across the component tree.
const FormContext = createContext<FormGeneratorContextType | undefined>(undefined);
Form Component
The Form component initializes a form using Mantine's useForm hook and renders the form with its children. It also provides a context provider for the form-related context.
export const Form = ({ onSubmit, initialValues, validations, children, customForm, handleError }: FormProps) => {
const form = useForm({
initialValues: initialValues,
validate: validations,
});
const formToUse = customForm || form;
return (
<FormContext.Provider value={{ form: formToUse, initialValues, validations }}>
<form onSubmit={formToUse.onSubmit(onSubmit, handleError)}>{children}
</FormContext.Provider>
);
};
Form Field Component
The Form.Field component is used to render different types of form fields based on the field configuration. It uses Mantine components like TextInput, Select, DatePicker, etc., to render the appropriate input based on the specified type in the FieldConfig.
Form.Field = ({ field }: { field: FieldConfig }) => {
const context = useContext(FormContext);
if (!context) {
throw new Error("FormGenerator.Inputs must be used within a FormGenerator");
}
const { form } = context;
const renderInput = useMemo(() => {
let formProps: any;
switch (field.type) {
// ... (Switch case for different field types)
}
}, [field, form]);
return <>{
{renderInput}}</>;
};
To apply the above code structure to a Vehicle Fleet Management scenario, let's make some adjustments and create a simplified example. In this example, we'll consider fields relevant to vehicle management, such as vehicle name, type, registration number, and maintenance status.
Types for Vehicle Fleet Management
interface Vehicle {
name: string;
type: string;
registrationNumber: string;
maintenanceStatus: boolean;
}
interface VehicleFieldConfig extends FieldConfig {
type: "text" | "select" | "switch";
}
interface VehicleFormProps extends FormProps {
onVehicleSubmit: (vehicle: Vehicle) => void;
}
Context Usage
// Assuming you have a FleetContext that manages the overall fleet data
const FleetContext = createContext<YourFleetContextType | undefined>(undefined);
Certainly! To apply the provided code structure to a Vehicle Fleet Management scenario, let's make some adjustments and create a simplified example. In this example, we'll consider fields relevant to vehicle management, such as vehicle name, type, registration number, and maintenance status.
Types for Vehicle Fleet Management:
jsxCopy codeinterface Vehicle { name: string; type: string; registrationNumber: string; maintenanceStatus: boolean; } interface VehicleFieldConfig extends FieldConfig { type: "text" | "select" | "switch"; } interface VehicleFormProps extends FormProps { onVehicleSubmit: (vehicle: Vehicle) => void; }
Context Usage:
jsxCopy code// Assuming you have a FleetContext that manages the overall fleet dataconst FleetContext = createContext<YourFleetContextType | undefined>(undefined);
Form Component for Vehicle Fleet Management
export const VehicleForm = ({ onVehicleSubmit }: VehicleFormProps) => {
const fleetContext = useContext(FleetContext);
if (!fleetContext) {
throw new Error("VehicleForm must be used within a FleetContextProvider");
}
const form = useForm({
initialValues: {}, // Initial values for the vehicle form
validate: undefined, // You can add validations specific to vehicles if needed
});
return (
<FormContext.Provider value={{ form }}>
<form onSubmit={form.onSubmit((values) => onVehicleSubmit(values as Vehicle))}>
{/* Render Vehicle form fields using Form.Field component */}
<Form.Field field={{ type: "text", label: "Vehicle Name", name: "name", defaultValue: "" }} />
<Form.Field field={{ type: "select", label: "Vehicle Type", name: "type", defaultValue: "", options: ["Car", "Truck"] }} />
<Form.Field field={{ type: "text", label: "Registration Number", name: "registrationNumber", defaultValue: "" }} />
<Form.Field field={{ type: "switch", label: "Maintenance Status", name: "maintenanceStatus", defaultValue: false }} />
<button type="submit">Submit Vehicle</button>
</form>
</FormContext.Provider>
);
};
Hope this article on Form validations in vehicle fleet management is useful to you. Please read Authentication in fleet management